Link of the day: http://sauerbraten.org/
Sauerbraten (a.k.a. Cube 2) is a free multiplayer/singleplayer first person shooter, built as a major redesign of the Cube FPS.
Monday, 07 May 2007
Friday, 04 May 2007
First Model
Thursday, 03 May 2007
Rocket Commander

David blogged about this in his blog and I just want to reiterate it:
Rocket Commander is a... well... interesting little game that, personally, I found pretty boring as far as gameplay is concerned. However, from a technical-learning-games-programming point of view it is a goldmine of useful information. The game was written by Benjamin Nitschke and is free--with source code.
There's a very informative page on MSDN that has a few tutorials on Rocket Commander. Check it out--they're really, really useful.
Now I just gotta get my hands on Benjamin's book.
Tuesday, 01 May 2007
Bullets
I've been playing around a lot and browsing copious amounts XNA tutorials. Some of them amazing, some of them outright lame (I'm not going to link the lame ones).
I figured out how I'm going to do gamestate. I've tried a number of different ways and the one that I think works best is to maintain a stack of "GameScreen" objects. Each game screen is loaded on the top of the stack and disables the one before it. As the screen is dismissed, it reveals the previous one.
I have a working copy--screenshot won't show much though:
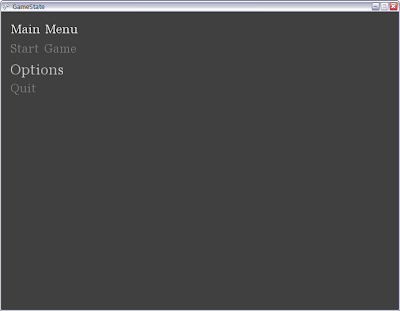
I've also been playing around with additive transparency on sprites. Most notably, for glowy bullets. I managed to get bullets but they're not really "glowy":
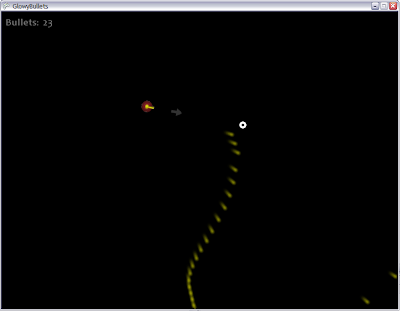
Oh, and ArcTan(x/y)--how I hate thee. Turns out that if you divide a negative by a negative then you get a positive--who would have guessed *grin*.
I had to do this hacky check to get my firing angle to work:
And with that, it's off to bed.
I figured out how I'm going to do gamestate. I've tried a number of different ways and the one that I think works best is to maintain a stack of "GameScreen" objects. Each game screen is loaded on the top of the stack and disables the one before it. As the screen is dismissed, it reveals the previous one.
I have a working copy--screenshot won't show much though:
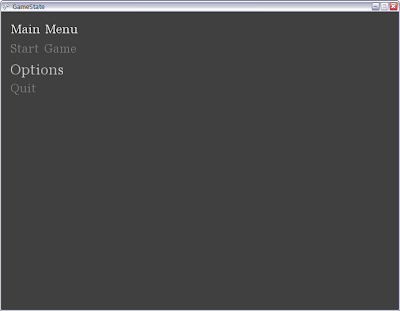
I've also been playing around with additive transparency on sprites. Most notably, for glowy bullets. I managed to get bullets but they're not really "glowy":
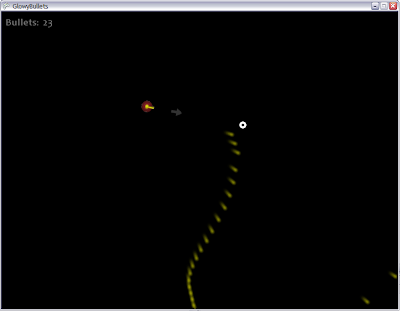
Oh, and ArcTan(x/y)--how I hate thee. Turns out that if you divide a negative by a negative then you get a positive--who would have guessed *grin*.
I had to do this hacky check to get my firing angle to work:
firingAngle = (float)(Math.Atan((double)y / (double)x));
if (x < 0) firingAngle += MathHelper.Pi;
And with that, it's off to bed.
Wednesday, 25 April 2007
Transparent textures with MDX
This is just a quick, late night writeup on how to draw transparent textures onto the screen using Managed DirectX.
Firstly, we load the texture in an initilisation routine:
Then we render the texture onto a 2-triangle trianglefan:
The important things to notice are:
This is what the final product looks like:
Firstly, we load the texture in an initilisation routine:
public void Initialize()
{
tex = TextureLoader.FromFile(Form1.Device, ".\\test.png");
rect = new Rectangle(50, 50, 40, 40); // assuming our png is 40x40
}
Then we render the texture onto a 2-triangle trianglefan:
public void Render()
{
// device has been cleared and beginscene has been called
CustomVertex.TransformedTextured[] screenVertices =
new CustomVertex.TransformedTextured[4];
screenVertices[0] = new CustomVertex.TransformedTextured(
rect.X, rect.Y, 1.0f, 1.0f, 0.0f, 0.0f);
screenVertices[1] = new CustomVertex.TransformedTextured(
rect.X + rect.Width, rect.Y, 1.0f, 1.0f, 1.0f, 0.0f);
screenVertices[2] = new CustomVertex.TransformedTextured(
rect.X + rect.Width, rect.Y + rect.Height, 1.0f, 1.0f, 1.0f, 1.0f);
screenVertices[3] = new CustomVertex.TransformedTextured(
rect.X, rect.Y + rect.Height, 1.0f, 1.0f, 0.0f, 1.0f);
Form1.Device.RenderState.SourceBlend = Blend.SourceAlpha;
Form1.Device.RenderState.DestinationBlend = Blend.InvSourceAlpha;
Form1.Device.SetRenderState(RenderStates.AlphaBlendEnable, true);
Form1.Device.SetTexture(0, tex);
Form1.Device.VertexFormat = CustomVertex.Transform
edTextured.Format;
Form1.Device.DrawUserPrimitives(PrimitiveType.TriangleFan, 2, screenVertices);
// endscene and present will be called by main render loop
}
The important things to notice are:
- RenderState.SourceBlend = Blend.SourceAlpha
- RenderState.DestinationBlend = Blend.InvSourceAlpha
- SetRenderState(RenderStates.AlphaBlendEnable, true)
This is what the final product looks like:
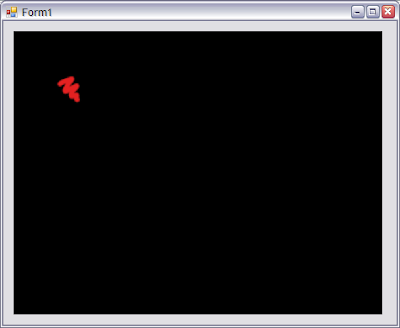
Tuesday, 24 April 2007
MDX-101: A simple window
To get a simple DirectX window running inside a panel on a form using Managed DirectX do the following:
1) Create a new WinForms project
2) On the main form, drop a panel (panel1) onto the form.
3) Change the Main() function in Program.cs to look as follows:
4) Put the following code into Form1.cs:
Don't forget to reference Microsoft.DirectX.
That's it.
1) Create a new WinForms project
2) On the main form, drop a panel (panel1) onto the form.
3) Change the Main() function in Program.cs to look as follows:
static void Main()
{
using (Form1 frm = new Form1())
{
frm.Show();
while (frm.Created)
{
frm.Render();
Application.DoEvents();
}
}
}
4) Put the following code into Form1.cs:
private Device device;
public Form1()
{
InitializeComponent();
InitDirectX();
}
void InitDirectX()
{
PresentParameters pp = new PresentParameters();
pp.SwapEffect = SwapEffect.Discard;
pp.Windowed = true;
device = new Device(0, DeviceType.Hardware, panel1,
CreateFlags.SoftwareVertexProcessing, pp);
}
internal void Render()
{
device.Clear(ClearFlags.Target, Color.Black, 1.0f, 0);
device.BeginScene();
device.EndScene();
device.Present();
}
Don't forget to reference Microsoft.DirectX.
That's it.
XNA Refresh Available
The Microsoft XNA Game Studio Express 1.0 Refresh is now available.
Note that the update requires:
If you're upgrading from the 1.0 version of XNA Game Studio Express you do not need to uninstall that version first.
You can download the XNA Framework Redistributable here.
Note that the update requires:
- Microsoft Visual C# 2005 Express Edition SP1
- That you run C# 2005 Express Edition at least once prior to installing the XNA Game Studio Express.
- That you register C# 2005 Express Edition prior to installing the XNA Game Studio Express.
If you're upgrading from the 1.0 version of XNA Game Studio Express you do not need to uninstall that version first.
You can download the XNA Framework Redistributable here.
Subscribe to:
Posts (Atom)